Creating a Shipping Method in Magento 2
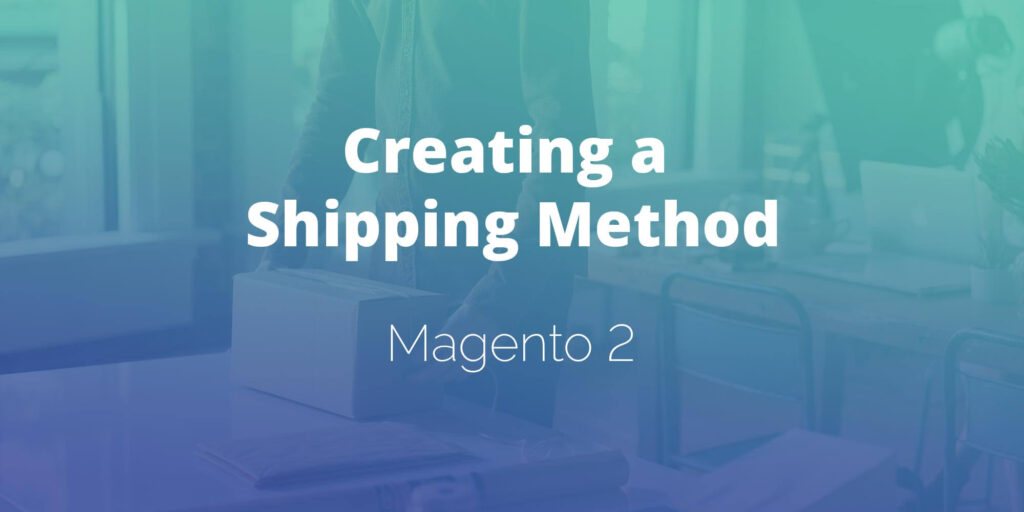
There are existing extensions available for many of the shipping carriers that you may choose to utilize on your Magento 2 site, but what about a shipping method of your own? Adding a custom shipping method like this is a straightforward approach that requires only a handful of files in order to implement – five, […]
A Look at CSS and Less in Magento 2
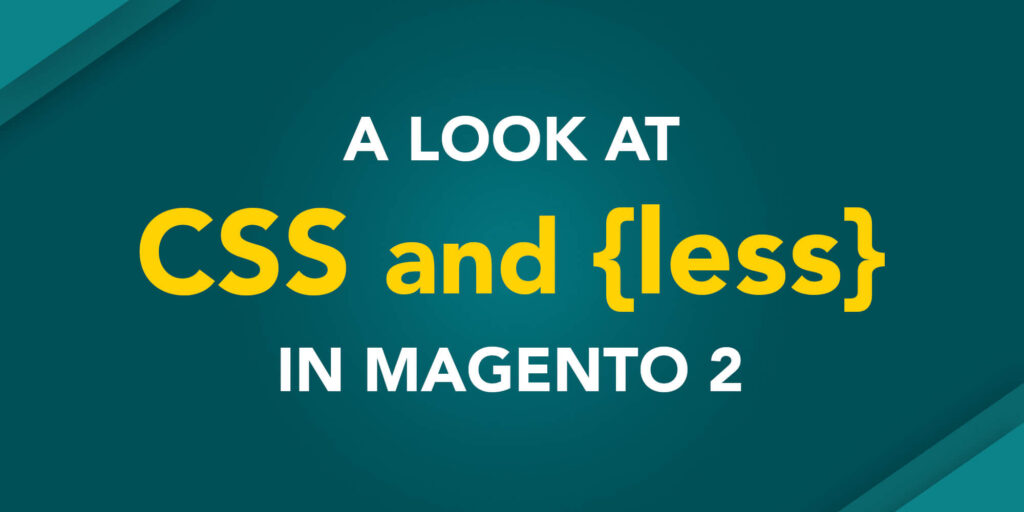
Magento 2 leverages the enormous power of CSS pre-processing (via Less) to make theme customization intuitive and easily maintainable through features like variables, mixins, and imports. Less compilation is a fundamental part of M2’s static content deployment and development tools, streamlining the theming process and ensuring that frontend developers can focus on their code, not […]
Canonical Tags: Did You Get It Right on Your Magento Site?
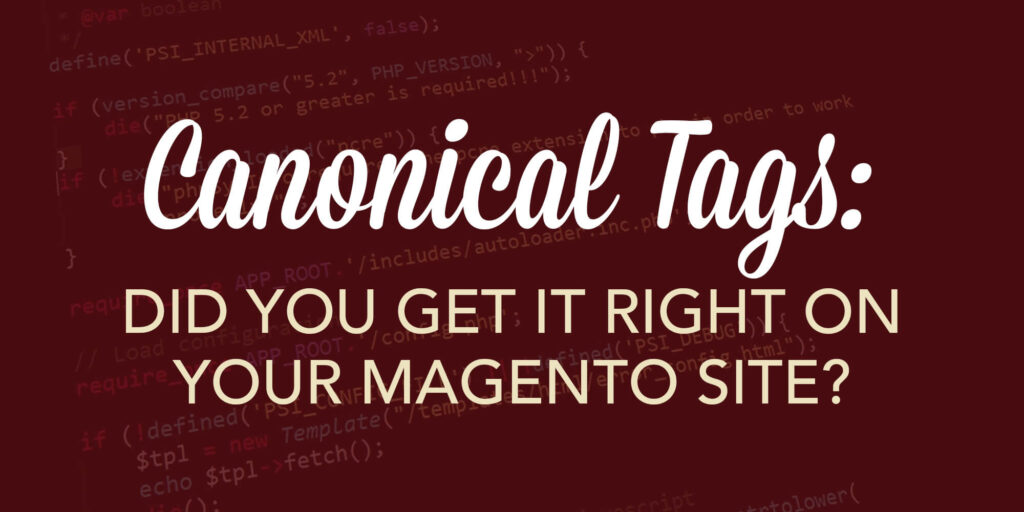
A few weeks ago, a llama posed a seemingly simple question: “Does anyone know the details of canonical URLs and pagination?”. But here’s the thing about llamas – we don’t do well with non-definitive answers. Thus began the digging and twists and turns into the rabbit hole of canonical tags and Magento’s native capabilities. Here […]
User Acceptance Testing a Magento 2 Site
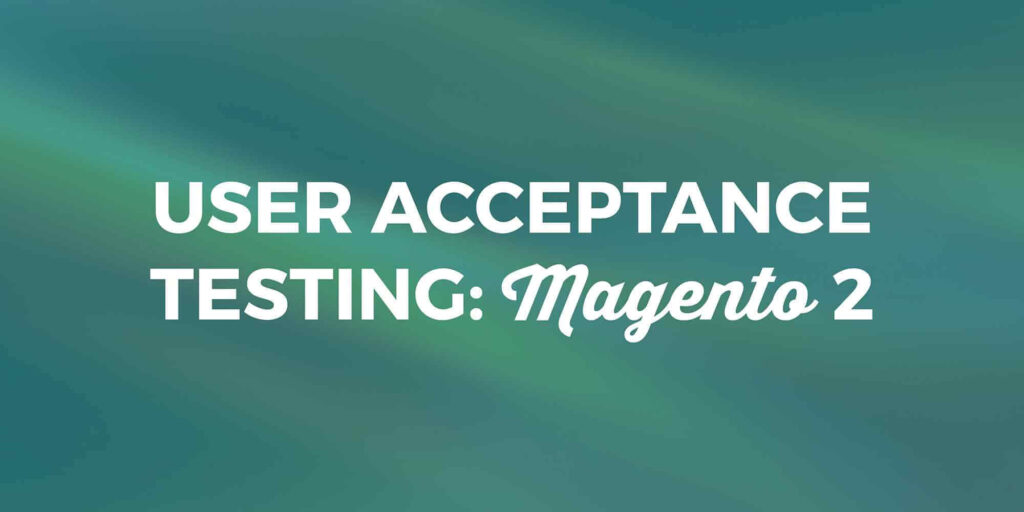
During our website builds, some of our clients have asked about our quality assurance process. We commonly get questions like “What do you check during testing?” and “What should our testing include?” We can give answers to the first question during the course of the project. In response to the second question, we recently wrote […]
Google Analytics and Magento 2
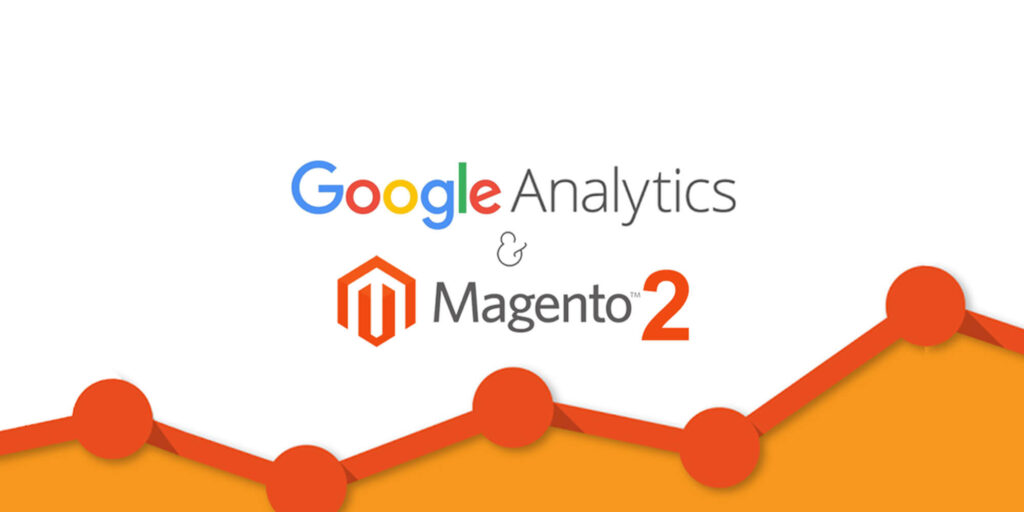
Google Analytics is an important tool for merchants in the eCommerce and Magento communities because it allows merchants to make informed decisions about their website based on their users’ interactions. With the advent of Magento 2, it’s important to understand how the new version utilizes and integrates with Google Analytics. If you’re familiar with Magento […]
Maintaining Excellent Customer Service at Scale for R&R Products, Inc.
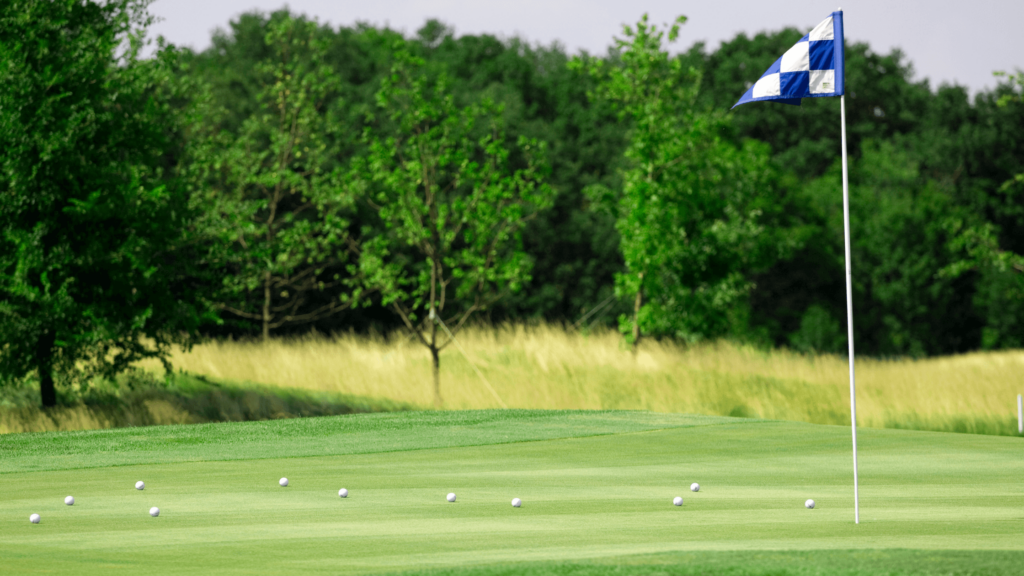
Sometimes, no matter your best intentions, a project can be laden with difficulties. That was certainly true for the R&R Products, Inc., company in the early stages of their eCommerce journey. When the R&R team decided their new goals were to expand from the golf products industry into the landscaping market, all while keeping up […]